My new game, Rightwaves, is out now! It’s a tribute to the great classic R-Type.

The entire implementation, including all the graphics, is contained within a single ~5000-line JavaScript file. Why?
This is a terrible idea
Let me start by saying I do not recommend writing code this way. It’s a terrible idea, and some aspects of the development process have been severely hampered by doing this.
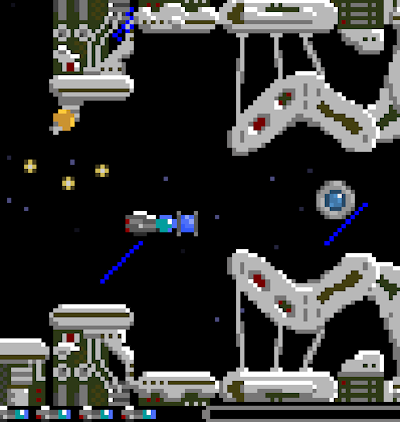
Complexity
One of my goals for Smolpxl is to strip coding back to the simplest possible thing it can be.
Over the last 40 years, programming has changed a huge amount: the number and variety of the tools we have to work with has increased beyond what I can take in, and almost all of these things are utterly brilliant.
I can’t imagine writing a game like Eat Apples Quick using the line-orientated editor I used on the ZX Spectrum. I thoroughly enjoyed the Rust code completion and documentation I get from running rust-language-server inside NeoVim. (I’ve even heard there are editors that work inside a windowed environment, but I am not brave enough to try one.)
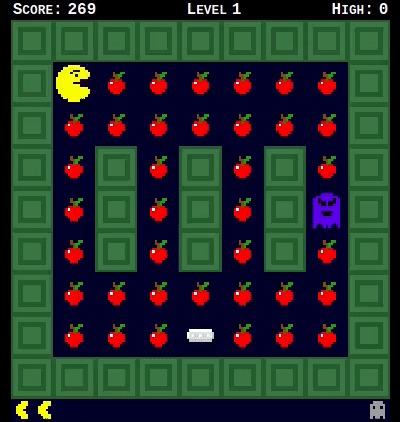
I can’t imagine writing the simple physics engine for Spring in assembly language, or trying to concentrate on that while I had to unravel complex graphics rendering conventions and timings. Instead, I was able to write code that looked a bit like the maths it represented, and allow the browser to handle the graphics card.

But, along with all these powerful tools like high-level programming languages, fully cross-platform user interface components, and libraries that make writing a game loop simple, comes a new problem: complexity.
We must choose which tools, libraries and paradigms we’re going to use, and to make that choice we need to understand them.
The Smolpxl library makes some of these choices for you, by enforcing an Elm-style model/update/view split, providing a pixellated canvas of known size, and handling a game loop with fixed framerate. This is particularly suited to writing little retro-style games, similar to what I used to write in AMOS Basic on my Amiga.
I want to make everything simple, but I want our game to work on people’s computers. The only platform we can realistically choose is the browser: the exact same code works almost everywhere.
None of this explains why I would write a game inside a single file of source code, but hopefully it begins to demonstrate where I am coming from.
Simplicity
Another goal for Smolpxl is to teach programming, and a huge barrier I see to getting started is the build process.
No build
You can write Smolpxl games in Rust and compile them to WASM (which is how Eat Apples Quick is done), and you could also package the JavaScript with webpack or similar, but I want the first-class way of using Smolpxl to involve no build at all: I want you to be able to copy in a standard index.html and smolpxl.js and then write your code in game.js and have it just work when you double-click on index.html.
A goal for Rightwaves is to demonstrate that you can write a full game in Smolpxl, without stepping outside that simple story: just double-click on index.html.
It’s all inside game.js
So, Rightwaves is a single* JavaScript file containing the code, the level descriptions, and, probably most unusually, the graphics.
* Note: I cheated – the “action-replay” data is in a separate file – it was much bigger than the source code, and would have made it too hard to deal with. If I wanted to stay pure, I would have had to remove the default action replay from the game, but I just liked it too much.
Level design
Many times while I was writing Rightwaves, I wished I had written a level editor. In fact, I am often quoted as saying
“A game without a level editor is only half a game.”
— Andy Balaam, quite often
But instead, the level design is code like this:
const LEVELS = [
{
scenery: [
{ x: 0, y: 80, image: "machinery-20x16-01"},
{ x: 20, y: 88, image: "machinery-20x08-01"},
// ... etc.
],
width: 1526,
aliens: [
newRedFlat(130, 20),
newRedFlat(145, 25),
// ... etc.
The full code is at gitlab.com/smolpxl/rightwaves/-/blob/main/public/game.js#L3478.
It’s not a lot of fun to edit, but on the other hand, every time you make a change a simple refresh in the browser lets you see what it really looks like. No build process; no waiting.
Graphics
I drew the graphics for Rightwaves using GIMP, exported the images as PNG files, and converted them to text using a little Rust utility I wrote.
When I wanted to change an image, it was a nightmare, and I would recommend this approach to no-one whatsoever.
The reason why Smolpxl supports creating images in the source code is so you can hand-craft them right there, using an ASCII-art style.
Here’s the spaceship: 
and here is the code for it:
const IMAGES = {
"ship": {
pixels: [
"..www.......",
"dddddww.bbb.",
"rllldddcccwb",
"dddhlllccccb",
"rddddddaccb.",
"..aaa......."
],
key: {
"w": [255, 255, 255],
"d": [88, 88, 88],
"b": [77, 111, 249],
"r": [141, 0, 0],
"l": [126, 125, 125],
"h": [192, 192, 192],
"c": [3, 157, 157],
"a": [42, 42, 42]
}
},
// ... lots, and I do mean lots, more here for the other graphics ...
The full code is at gitlab.com/smolpxl/rightwaves/-/blob/main/public/game.js#L41.
Note that I painstakingly coloured that code in for you, for this blog post. In the code there is no such luxury!
Openness
All the Smolpxl games are Free/Open Source software, of course. By avoiding a build, anyone can see the source code, just as I wrote, it in their browser. Anyone (including me) can debug problems without any extra steps. Putting everything in one file makes it easier to find the code (but probably makes it harder to understand).
Optimisation
Rightwaves loads really fast. On my machine, the first time you visit the page, it is playing within 1.4 seconds, after downloading 5 files at a total of 149kB (gzipped). If we exclude the included action replay, it is much smaller.
All of this, with no build process.
Maybe it wasn’t such a bad idea after all.
This is a bad idea
Don’t try this.
For fun
Except, of course, if you like fun. I tried this because it was fun. I would heartily recommend trying things because they might be fun.
Also, if you like fun, try playing the games on Smolpxl games, or write your own!
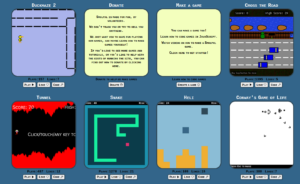